📑 readMessage()
The readMessage()
function in Easy-Yopmail allows you to read the content of a specific email within a YOPmail inbox. It provides options to retrieve the content in plain text or HTML format, and even allows you to target specific elements within the email using CSS selectors.
Input Parameters
Parameter | Type | Description |
---|---|---|
| String | The YOPmail email address (without the @yopmail.com domain). |
| String | The unique ID of the email you want to read. |
| Object | (Optional) An object with additional options to customize the read operation. |
Options within the options parameter:
format
: (Optional) Format of the email content. Can be "TXT" (plain text) or "HTML" (HTML). Defaults to "TXT".selector
: (Optional) A CSS selector to target a specific element within the HTML email body.attribute
: (Optional) If a selector is provided, this parameter specifies the attribute of the element to be returned (e.g., "href" for a link).pathToSave
: (Optional) Path to save the email HTML as a file.saveAttachments
: (Optional) Boolean flag to save email attachments to the project download folder.
Output
The readMessage()
function returns a promise that resolves with an object containing the following information:
id
: The ID of the email.submit
: The subject of the email.from
: The sender's email address.date
: The date the email was received.deliverability
: Information about the email delivery.attachments
: An array of attachment objects (ifsaveAttachments
is enabled).saveAttachments
: A boolean flag indicating if attachments were saved.format
: The format of the returned content ("TXT" or "HTML").selector
: The CSS selector used (if provided).eq
: The index of the selected element (if provided).attribute
: The attribute of the selected element (if provided).pathToSave
: The path where the HTML was saved (if provided).content
: The content of the email in the specified format.info
: A list of warnings or informational messages.
Mermaid Diagram
Practical Use Cases
1. Read Plain Text Content of an Email
2. Extract Confirmation Link from HTML Email
3. Save Full HTML Content of an Email to a File
4. Read Email Content and Save Attachments
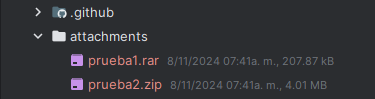